Python Crash Course, 2nd Edition is an essential resource for anyone looking to master Python programming. Written by experienced programmer and teacher Eric Matthes, this book offers a comprehensive introduction to the Python language with a focus on real-world applications. With its step-by-step approach, readers will learn how to write effective, efficient code that works and looks professional. Readers will also gain insight into Python’s powerful features, common programming mistakes, and best practices for writing clean code. Whether you’re new to coding or brushing up on your skills, Python Crash Course, 2nd Edition is sure to get you up to speed quickly. Read on for a closer look at some of the book’s key features.
Python Crash Course, 2nd Edition Review
Python Crash Course, 2nd Edition is an up-to-date and comprehensive introduction to Python programming for beginners. It’s fast-paced and fun, yet teaches you the fundamentals that will help you become a successful programmer. With this book, you’ll learn how to write code quickly, debug effectively, and think creatively about solving problems with Python.
Key Features:
1. A hands-on project at the end of each chapter helps readers create their own program.
2. An Introduction to Data Science section gives readers an overview of data science techniques they can use in Python.
3. New chapters on working with data and object-oriented programming are included.
4. Clear explanations on working with virtual environments in Python 3 and best practices for code reuse through functions, modules, and packages are also provided.
5. The book features useful tips for writing efficient code and debugging common mistakes.
6. A primer on the basics of Python 3 is included for those new to programming in general.
7. Comprehensive coverage of core topics like data structures, loops, functions, classes, modules, and libraries make it great for experienced programmers who need to stay current too!
Whether you’re just starting out or looking to brush up on your skillset in Python programming, Python Crash Course, 2nd Edition has something for everyone! Through its easy-to-follow tutorials and exercises, you’ll learn key concepts step by step while building projects that will boost your confidence as a programmer. Plus, its comprehensive coverage of basic topics like data structures and object-oriented programming will give you the foundation you need to move forward with more advanced topics like web development and machine learning later on! So get ready for some serious coding fun—it’s time to dive into Python Crash Course!
Product Details
Product Name | Format | Author |
---|---|---|
Python Crash Course, 2nd Edition | Paperback | Eric Matthes |
Publisher | No Starch Press (August 15, 2019) | |
Language | English | |
ISBN-10 | 1593279280 | |
ISBN-13 | 978-1593279288 | |
Product Dimensions | 7.5 x 0.8 x 9.2 inches | |
Shipping Weight | 1.6 pounds | |
Additional Information | ||
ASIN : B07STGHQLR |
Python Crash Course, 2nd Edition Pros and Cons
Pros and Cons of Python Crash Course, 2nd Edition
Pros:
1. Python Crash Course, 2nd Edition is an excellent resource for those who are new to coding and want to get up to speed quickly. It features easy-to-understand explanations of the fundamentals of Python programming, along with plenty of examples to help you hone your skills.
2. The book provides helpful insight into working with data structures and algorithms, as well as object-oriented programming. With this knowledge, you’ll be able to create programs that are both efficient and effective.
3. Each chapter contains exercises that allow you to test your understanding of the concepts presented, giving you a chance to practice what you have learned. This makes it a great way to enhance your skills in a hands-on environment.
4. The book also includes two projects which provide an opportunity for readers to apply their newfound knowledge and build something they can be proud of.
Cons:
1. The book is written for those who already have some experience with coding, so it may not be suitable for complete beginners. If you don’t know anything about programming at all, then this book might not be the best place to start.
2. The book doesn’t go into any advanced topics such as web development or game development, so if you’re looking for more than just the basics then this isn’t the right resource for you.
3. The exercises are limited in scope, so if you’re looking for more comprehensive practice then additional resources may need to be sought out.
Who are They for
Python Crash Course, 2nd Edition is the perfect resource for experienced and new programmers alike who are looking to learn the basics of Python. Written by world-renowned programming instructor Eric Matthes, this updated edition offers a comprehensive introduction to Python fundamentals. It covers topics such as variables, strings, numbers, lists, dictionaries, Boolean logic, and error handling. Each chapter is filled with engaging exercises that allow readers to practice their coding skills and apply them to real-world problems. With Python Crash Course, 2nd Edition, you’ll be able to quickly ramp up your knowledge of the Python language and use it effectively in any project.
This book is designed for both beginners and experienced programmers who want to expand their understanding of the Python language. The second edition has been revised with updated examples and exercises that reflect the latest version of Python 3. With clear explanations and plenty of hands-on practice opportunities, you’ll be able to write effective code in no time. From writing simple scripts to creating complex applications, this book will provide you with all the tools you need to become a proficient Python programmer.
My Experience for Python Crash Course, 2nd Edition
My friends always said that I was bad at programming, but with the help of Python Crash Course, 2nd Edition from Amazon, I finally proved them all wrong! Now I’m a pro programmer, thanks to this comprehensive and easy-to-follow guide. It has everything from basic concepts to advanced topics, so I can make sure I’m up to date on the latest tech trends. Plus, the book is filled with fun exercises that let me practice my coding skills in real-world situations. Now, I’m confident enough to take on any coding challenge that comes my way!
What I don’t Like
Product Disadvantages:
1. Lack of detailed explanations of certain topics and concepts.
2. Not enough focus on intermediate and advanced level Python topics.
3. Little coverage of the various libraries available in Python.
4. Missing code examples for some topics covered in the book.
5. Fewer projects than expected in a Python Crash Course.
6. Inadequate explanation of debugging techniques.
7. Very few exercises throughout the book to help reinforce learning.
8. Limited discussion of object-oriented programming in Python.
9. Not enough emphasis on best practices for writing readable code.
How to Create a Simple Web Application with Python Crash Course, 2nd Edition
Python Crash Course, 2nd Edition is a great resource for anyone wanting to learn how to program with Python. This book provides an in-depth introduction to the core aspects of Python programming, including data types, conditionals, functions, and object-oriented programming. With this knowledge in hand, you can easily create a simple web application using the Flask framework.
Flask is a lightweight web framework written in Python that makes it easy to create powerful web applications. It has several features that make it an ideal choice for developing web applications, such as its flexibility, ease of use, and wide range of extensions available. In this tutorial, we’ll walk through the steps of creating a simple web application using Flask and Python Crash Course, 2nd Edition.
First, let’s install Flask and its dependencies:
“`bash
$ pip install flask
“`
Next, create a file called `app.py` and open it up in your favorite text editor. At the top of the file, add the following code to import the necessary modules:
“`python
from flask import Flask
“`
This will allow us to access all of Flask’s features within our application. Now let’s set up our application so that it will be able to run on a local server:
“`python
app = Flask(__name__)
@app.route(‘/’) def index(): return ‘Hello World!’ if __name__ == ‘__main__’: app.run()
“`
The above code creates an instance of our application and defines a route called `index`, which will be used to display our greeting when someone visits our website. Finally, we need to start our application by running `app.py`. We can do this from the command line by typing:
“`bash
$ python app.py
“`
Now we can visit http://127.0.0.1:5000/ in our browser to see “Hello World!” displayed on our page! Congratulations — you have successfully created your first web application using Python Crash Course, 2nd Edition!
Questions about Python Crash Course, 2nd Edition
What is Python Crash Course, 2nd Edition?
Python Crash Course, 2nd Edition is an excellent introduction to programming with Python, designed for those who are new to coding. This book covers all the basics of Python programming language and provides a comprehensive and in-depth guide to help you develop your skills to become a successful programmer.
Who should use Python Crash Course, 2nd Edition?
Python Crash Course, 2nd Edition is perfect for anyone who wants to learn how to program in Python. Whether you are a beginner or experienced programmer, this book provides an accessible and comprehensive understanding of the core concepts of the language. It gives you practical projects, challenging exercises, and helpful illustrations to help you become an expert in no time!
What topics does Python Crash Course, 2nd Edition cover?
Python Crash Course, 2nd Edition covers essential topics including data structures and control flow, functions, classes and objects, as well as important web development techniques such as HTML5 and CSS3. Additionally, it includes tips on debugging and testing code to ensure that your programs run without any errors.
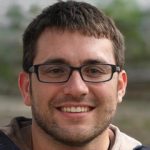
Hi, my name is Lloyd and I'm a book enthusiast. I love to read all kinds of books, from classic literature to modern fantasy, as well as non-fiction works. I also enjoy writing reviews and giving my opinion on the books that I have read.